This weekend I attended WordCamp Leipzig 2025, and WordCamp Leipzig is already a special one. It is super affordable, even for a WordCamp with just 9€ per ticket, this year tickets were in such high demand, that organizers sent out e-mails to ensure that people who bought a ticket were able to attend or pass on their ticket to people who didn’t get a ticket.
But that’s not all. Last year I got to know Manuela Grunert, or as she calls herself Yuri. We play Minecraft together and she’s blind, with about 2% of her eyesight remaining. And yes, 2% is legally blind in Germany, she’s still able to see colors and schematics. And if she’s close to things she can still read.
Since I’m always curious and had never had the chance to talk to a blind person before, I asked her if I could ask a few questions. She agreed. A few weeks later, I asked if she might want to share her insights with a broader group, perhaps at a WordPress Meetup, and that idea eventually grew into applying for WordCamp Leipzig.
The first talks by blind people at a German WordCamp
Her brother Mathias Grunert, who happens to be a blind developer, also applied, and both were chosen to speak in Leipzig. So we had not just one, but two talks by blind people in Leipzig.
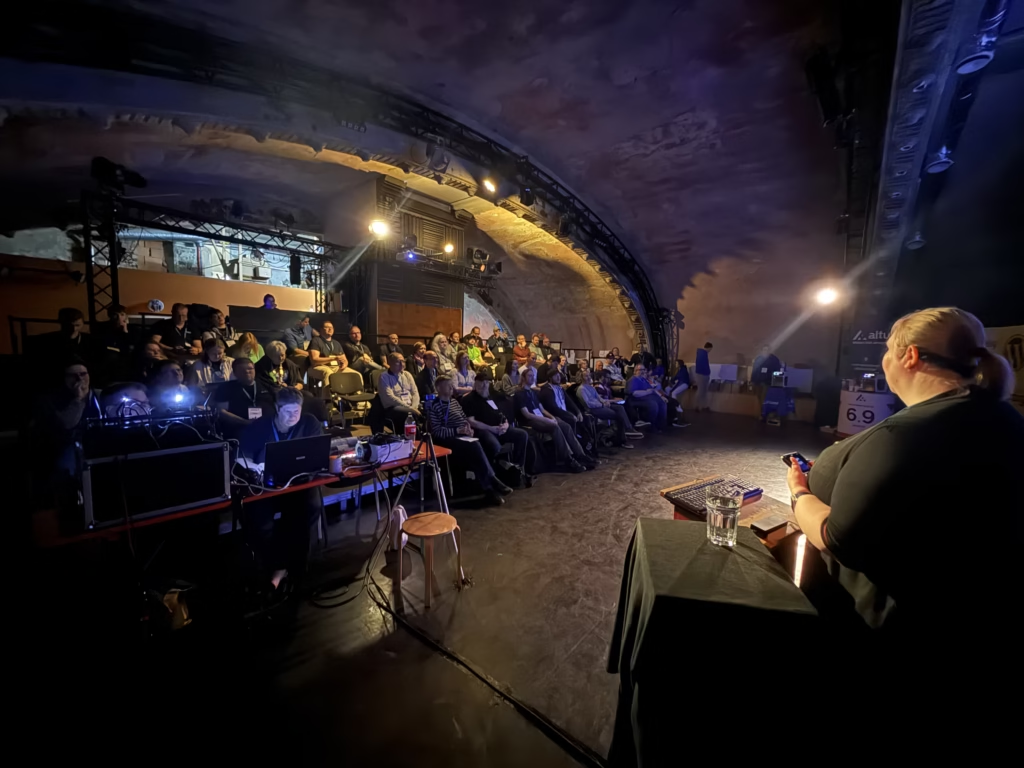
Yuri was very nervous, and I opted to be her MC, since I had thrown her in at the deep end. Both talks were great and – without any exaggeration or intended pun – eye-opening. It’s a whole different story when you hear from an accessibility expert about what you should do in theory, versus witnessing firsthand what happens if you don’t.
Yuri’s talk was about how she, as a user, uses the internet with a smartphone. And her opening questions, which we developed together, had the expected results and effect..
The audience of roughly 60 people was asked three questions and were told to lower their hand as soon as their answer was “No.” The first question was: “Who here has created or maintained a website?” – as expected, all hands remained up. The second question was: “Who checked it for accessibility?” – about 10 people lowered their hands. This was a little less than I expected, which was a positive surprise. But the third question had the expected result and impact: “And who of you did so on a smartphone?” – If I counted correctly, only 7 hands remained raised; everyone else, including myself, lowered their hands.
Yuri then presented her talk and hands-on examples using VoiceOver from her iPhone. For some, the VoiceOver was too fast; others appreciated seeing her actual personal experience. One audience member commented that some blind people listen to VoiceOver at up to 1,000 words per minute. For reference, you’ll probably read this article at about 200–240 words per minute.
The blind insighter – the second talk
Mathias had a different point of view – or point of experience? – as a developer he showed crafted examples that he came across over the years. My personal highlights in his talk: he presented his slides from memory, so the first roughly 10 minutes were done literally and figuratively blind. And during his hands-on session he did a few examples immersing us into his world, turning the screen black. For the given examples, none of us correctly guessed what VoiceOver tried to tell us.
Mathias announced that he would share these examples. As soon as I have the link, I will add it here.
My personal blind experience
Since I invited them, I also took care of getting them from the main station to the hotel, to the venue, and back. I also supported them during the WordCamp when needed.
For me, it was a humbling experience. Things that are a minor inconvenience for us, sighted people, can be a real struggle for Yuri, Mathias, and other visually impaired people.
A tram/subway/train without accurate announcements? For us, it’s annoying. For them? They might leave the train too early or miss their exit.
A steep staircase? A loose handrail? A narrow ceiling without marking? For them way more likely to stumble or hit their heads.
A fast food restaurant with fancy names for their products, their menu animated on a TV and no handout version? For us, it’s mostly no issue-at worst, mildly annoying. They have to ask what’s on the menu.
I go home with a better understanding of their struggles in life and am impressed by what they achieve without senses that we take for granted.
Others also welcomed them with open arms into the community, and they have already been asked to speak at other WordCamps. So there is hope that this will not be their last appearance at WordCamps. And maybe it will encourage others to follow their example.
Call for speakers and organizers
We learned a lot from both of them, and in my humble opinion, witnessing it firsthand is extremely valuable-more valuable than I had imagined. A11y experts can only share theoretical knowledge. While we still need this, understanding why is a whole different story.
Impaired speakers
So if YOU are visually impaired, blind, deaf, have color blindness, or any other restriction that makes it harder for you to take part in everyday life on the internet, reach out to your local community-whether it’s a WordPress meetup, WordCamp, or similar communities for Joomla, TYPO3, Drupal, etc.-and share your story.
Organizers
The same goes for organizers of events: accessibility is becoming more and more important. If you know anyone, encourage them to share their personal experience. You can also reach out to me or to the organizers of WordCamp Leipzig.
Thank you Everyone Else
Even though I did not cover the remaining talks at WordCamp Leipzig, I also want to thank everyone else: the organizers, the speakers, and all attendees.
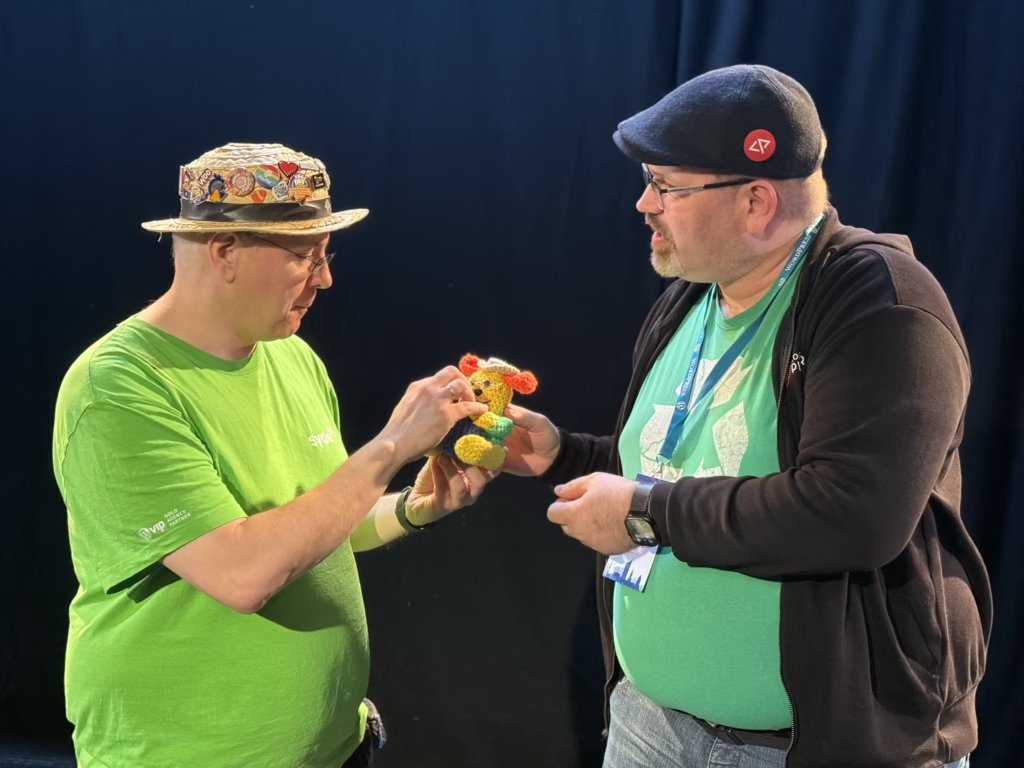